pass class test(): def __init__(self): pass def abc(): pass pass는 클래스나 함수를 완성하지 않은 상태에서 오류가 나지 않게 보류해두는 상태를 만듭니다. super class People: def __init__(self): print("People 생성자") class detailPeople(People): def __init__(self): #People.__init__(self) super().__init__() super를 사용해서 부모클래스에 접근이 가능합니다. 위의 코드처럼 부모 클래스 대신에 super로 사용이 가능하며, self도 생략이 가능합니다. super를 썼을때의 단점으로 다중상속을 받을 경우 super가 두 부모 클래스 중 어떤 클..
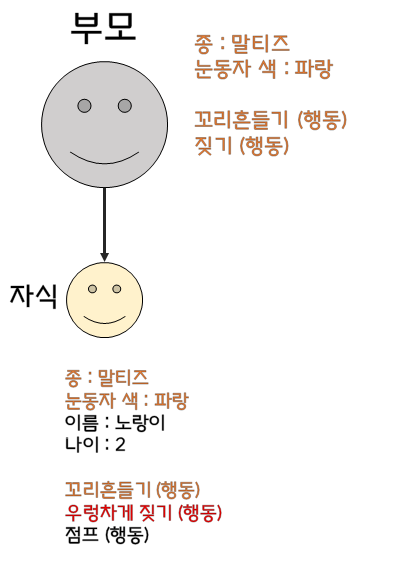
메소드 오버라이딩이 이미 선언되어있는 메소드를 상속받은 클래스에서 재정의해서 사용하는것을 의미합니다 위의 그림에서 메소드 오버라이딩은 부모로 부터 받은 메소드인 "짖기"를 새로 정의해서 우렁차게 짖기로 사용한 예입니다. class People: def __init__(self, name, age, weight, run_speed): self.name = name self.age = age self.weight = weight self.run_speed = run_speed print("{0}이 입장하였습니다.".format(self.name)) print("나이는 {0}, 몸무게는 {1} 입니다.".format(self.age, self.weight)) def move(self): print("{0}이 ..
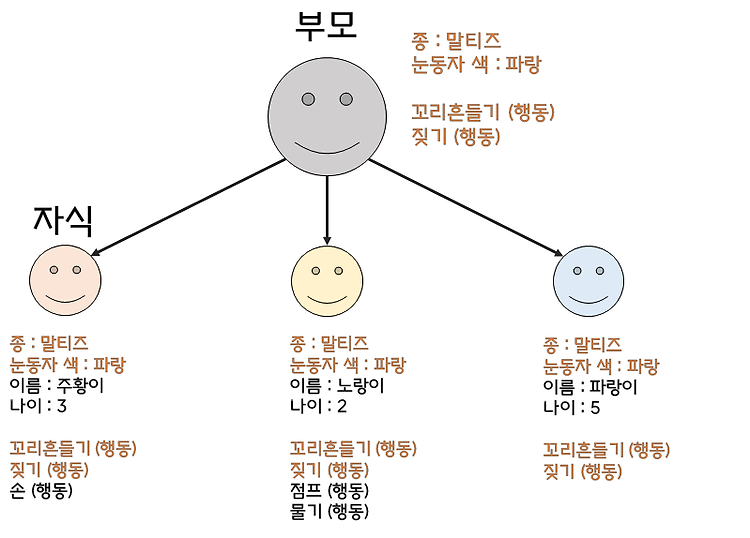
상속 - 부모 클래스의 변수와 메소드를 자식 클래스에서도 사용이 가능합니다 class People: def __init__(self, name, age, weight): self.name = name self.age = age self.weight = weight print("{0}이 입장하였습니다.".format(self.name)) print("나이는 {0}, 몸무게는 {1} 입니다.".format(self.age, self.weight)) class detailPeople(People): def __init__(self, name, age, weight, height): People.__init__(self, name, age, weight) self.height = height guest_3 = ..
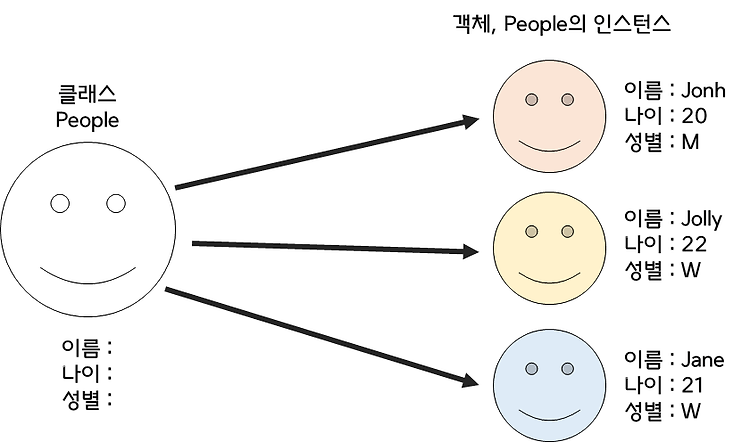
클래스는 변수와 메소드를 가지고 있습니다. 해당 클래스로 새로운 객체를 만들면 그 객체는 해당 클래스의 변수와 메소드를 소유하게 됩니다 클래스로부터 만들어지는 것을 객체라고 합니다. 아래에서 guest는 People 클래스의 인스턴스 입니다. __init__는 클래스의 생성자 역할을 합니다. class People: def __init__(self, name, age, weight): self.name = name self.age = age self.weight = weight print("{0}이 입장하였습니다.".format(self.name)) print("나이는 {0}, 몸무게는 {1} 입니다.".format(self.age, self.weight)) guest_1 = People("OWEN", ..
pickle 파일을 with를 통해 읽어들이는 방법 import pickle with open("test.pickle", "rb") as test_file: print(pickle.load(test_file)) with를 이용해 쓰고 읽는 방법 with open("python.txt", "w", encoding="utf8") as python_file: python_file.write("Hello, Python!") with open("python.txt", "r", encoding="utf8") as python_file: print(python_file.read())
텍스트 내용을 저장하거나,읽을 때는 파일 입 출력을 사용합니다. 하지만 리스트나 클래스처럼 자료형 자체를 저장하거나 읽을 때는 pickle 모듈을 사용해야합니다 import pickle test_file = open("test.pickle", "wb") test = {"A":1, "B":2, "C":["4", "5", 6]} print(test) pickle.dump(test, test_file) test_file.close() test_file = open("test.pickle", "rb") test = pickle.load(test_file) print(test) test_file.close() 출력 결과 {'A': 1, 'B': 2, 'C': ['4', '5', 6]} {'A': 1, 'B': 2..
open 함수를 통해서 파일을 열어줍니다. 첫번째 파라미터에서 대상을 적어주고, 두번째 파라미터에서 사용 방식을 적어줍니다. (r = read, w = write, a = append) encoding을 utf8로 지정해주는 것은 한글이 깨질 수 있기 때문입니다. test_file = open("test.txt", "w", encoding="utf8") print("Hello,", file=test_file) print("Python!", file=test_file) test_file.close() 파일을 w로 실행시켜서 print로 내용을 입력해보았습니다. w로 실행시킨 내용은 안에 내용이 있다면 덮어씌어지게 됩니다. 결과 내용은 아래의 내용을 가진 test.txt 파일이 생성되었습니다. Hello, ..
end를 설정해주면 print문 사용후 줄바꿈을 동작을 원하는 동작으로 바꿀 수 있습니다 print(2, end = " ") print(2, end = " ") 출력 결과 2 2 sep를 설정해주면 구분된 문자 사이에 설정된 값을 넣어줄 수 있습니다. print(2, 4, sep = "...") 출력 결과 2...4 import sys print("hello", file=sys.stdout) print("hello", file=sys.stderr) sys.stdout - 표준 출력으로 문장 출력 sys.stderr - 표준 에러로 문장 출력 로그 처리할 때 사용 dic = {"ABC":100, "DEF":110, "GHI":200} for alpha, num in dic.items(): print(alp..